How to use LINQ Query
What is LINQ?
LINQ (Language Integrated Query) is a Microsoft .NET Framework component that adds native data querying capabilities to .NET languages.
Getting Started with LINQ in C#
MongoDB .Net Driver LINQ Reference
What is LINQ Query Tool?
With LINQ Query Tool you can interactively query MongoDB database using LINQ.
Moreover you can execute any C# expressions and statements with this tool.
LINQ Query Tool is designed for C# developers. It is the best and the fastest way to create and check LINQ statements for your .Net projects.
How to use LINQ Query Tool?
1. To start LINQ Query Tool connect to a database and click Tools|LINQ Query in the main menu or LINQ Query icon on the toolbar.
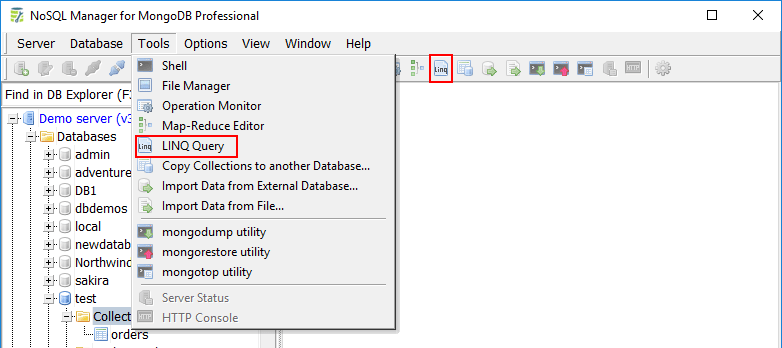
2. After that you see the following code snippet.
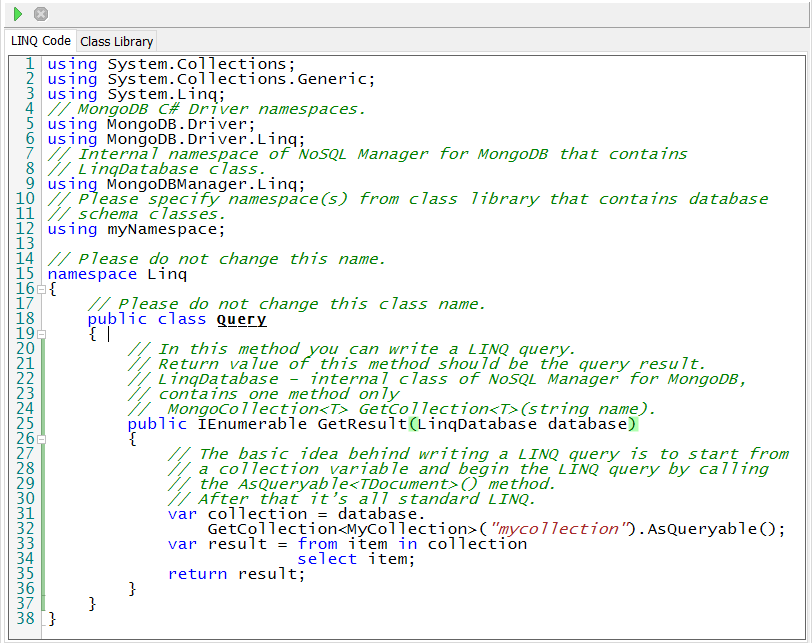
3. Next you need to specify .Net class library that defines your database structure.
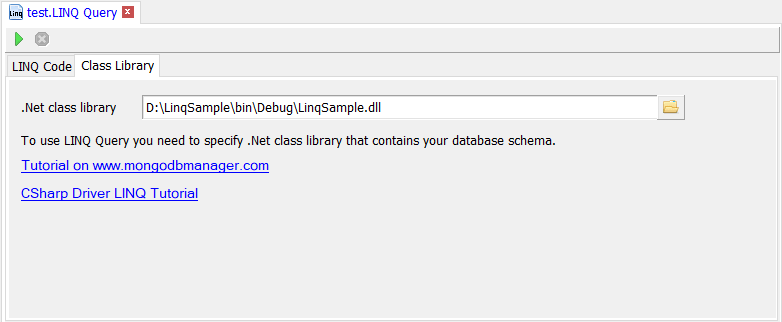
Please note: your schema classes should be declared as public, otherwise you will get the following error:
error CS0122: ‘SomeClass’ is inaccessible due to its protection level’ error
4. After that specify namespace there your classes are declared.
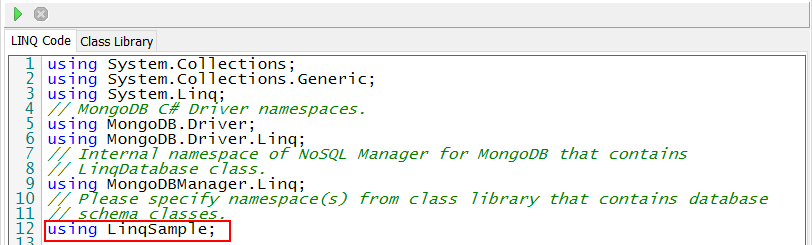
5. Next specify your class name, the corresponding collection name and a LINQ clause.
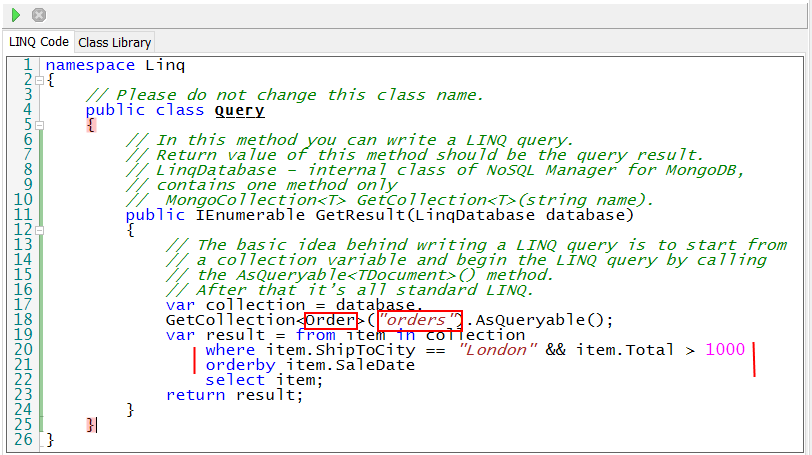
The basic idea is getting a reference to a collection variable in the usual way:
var collection = database.GetCollection<TDocument>(“collection_name”).AsQueryable();
where
database – internal class of NoSQL Manager for MongoDB, contains one method only:
public MongoCollection<T> GetCollection<T>(string name);
collection_name – name of your collection,
TDocument – name of a .Net class you want to map data to.
6. Just press F5 key to execute an expression and fetch result.
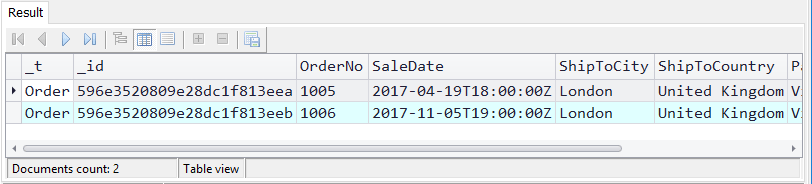
Example - Create simple LINQ query:
1. Connect to a database, open Shell and execute the script below to create orders collection:
db.createCollection("orders"); db.orders.insert( [{ "OrderNo" : NumberInt(1004), "SaleDate" : ISODate("2017-04-16T18:00:00Z"), "ShipToCity" : "Johannesburg", "ShipToCountry" : "Republic So. Africa", "PaymentMethod" : "Check", "ItemsTotal" : 7885 }, { "OrderNo" : NumberInt(1005), "SaleDate" : ISODate("2017-04-19T18:00:00Z"), "ShipToCity" : "London", "ShipToCountry" : "United Kingdom", "PaymentMethod" : "Visa", "ItemsTotal" : 4807 }, { "OrderNo" : NumberInt(1006), "SaleDate" : ISODate("2017-11-05T19:00:00Z"), "ShipToCity" : "London", "ShipToCountry" : "United Kingdom", "PaymentMethod" : "Visa", "ItemsTotal" : 31987 }, { "OrderNo" : NumberInt(1010), "SaleDate" : ISODate("2017-05-10T18:00:00Z"), "ShipToCity" : "Moscow", "ShipToCountry" : "Russia", "PaymentMethod" : "COD", "ItemsTotal" : 4996 }, { "OrderNo" : NumberInt(1059), "SaleDate" : ISODate("2017-02-23T19:00:00Z"), "ShipToCity" : "Kapaa Kauai", "ShipToCountry" : "America", "PaymentMethod" : "Cash", "ItemsTotal" : 2150 }] );
2. Next open LINQ Query, click on Class Library tab and specify the library below in the .Net class library field:
C:\Program Files (x86)\NoSQL Manager for MongoDB\Linq\LinqSample.dll
where C:\Program Files (x86)\NoSQL Manager for MongoDB\ is an installation folder of NoSQL Manager for MongoDB.
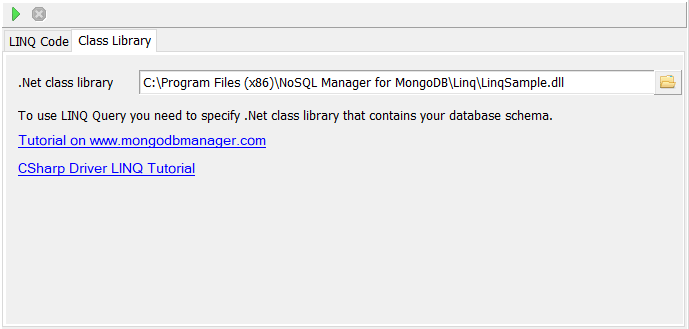
LinqSample.dll library contains one class used for mapping to the orders collection documents.
using System; using MongoDB.Bson; using MongoDB.Bson.Serialization.Attributes; namespace LinqSample { [BsonIgnoreExtraElements] public class Order { [BsonId] public ObjectId Id { get; set; } [BsonElement("OrderNo")] public int OrderNo { get; set; } public DateTime SaleDate { get; set; } public string ShipToCity { get; set; } public string ShipToCountry { get; set; } public string PaymentMethod { get; set; } [BsonElement("ItemsTotal")] public double Total { get; set; } } }
3. Next go to LINQ Code tab and paste the code below to the editor.
using System.Collections; using System.Collections.Generic; using System.Linq; // MongoDB C# Driver namespaces. using MongoDB.Driver; using MongoDB.Driver.Linq; // Internal namespace of NoSQL Manager for MongoDB that contains // LinqDatabase class. using MongoDBManager.Linq; // Please specify namespace(s) from class library that contains database // schema classes. using LinqSample; // Please do not change this name. namespace Linq { // Please do not change this class name. public class Query { // In this method you can write a LINQ query. // Return value of this method should be the query result. // LinqDatabase – internal class of NoSQL Manager for MongoDB, // contains one method only // MongoCollection GetCollection(string name). public IEnumerable GetResult(LinqDatabase database) { // The basic idea behind writing a LINQ query is to start from // a collection variable and begin the LINQ query by calling // the AsQueryable() method. // After that it’s all standard LINQ. var collection = database. GetCollection<Orders>("orders").AsQueryable(); var result = from item in collection where item.ShipToCity == "London" && item.Total > 1000 orderby item.SaleDate select item; return result; } } }
4. Press F5 key or click Execute button on toolbar.
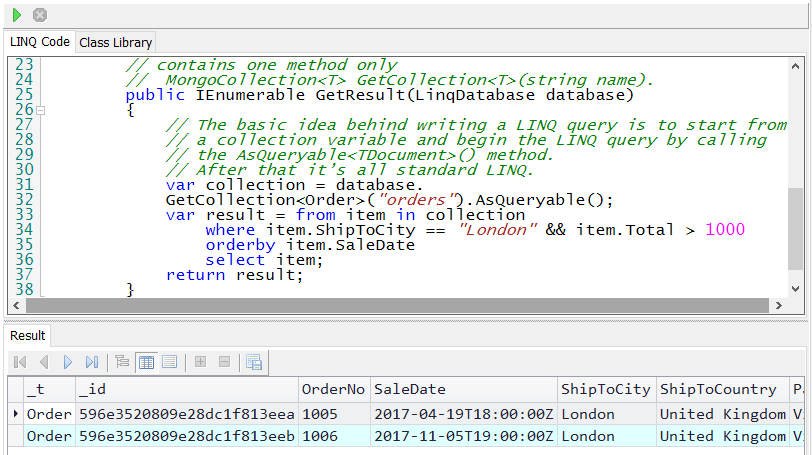